CRUD操作から見るAxiosとFetch APIの違いとは?
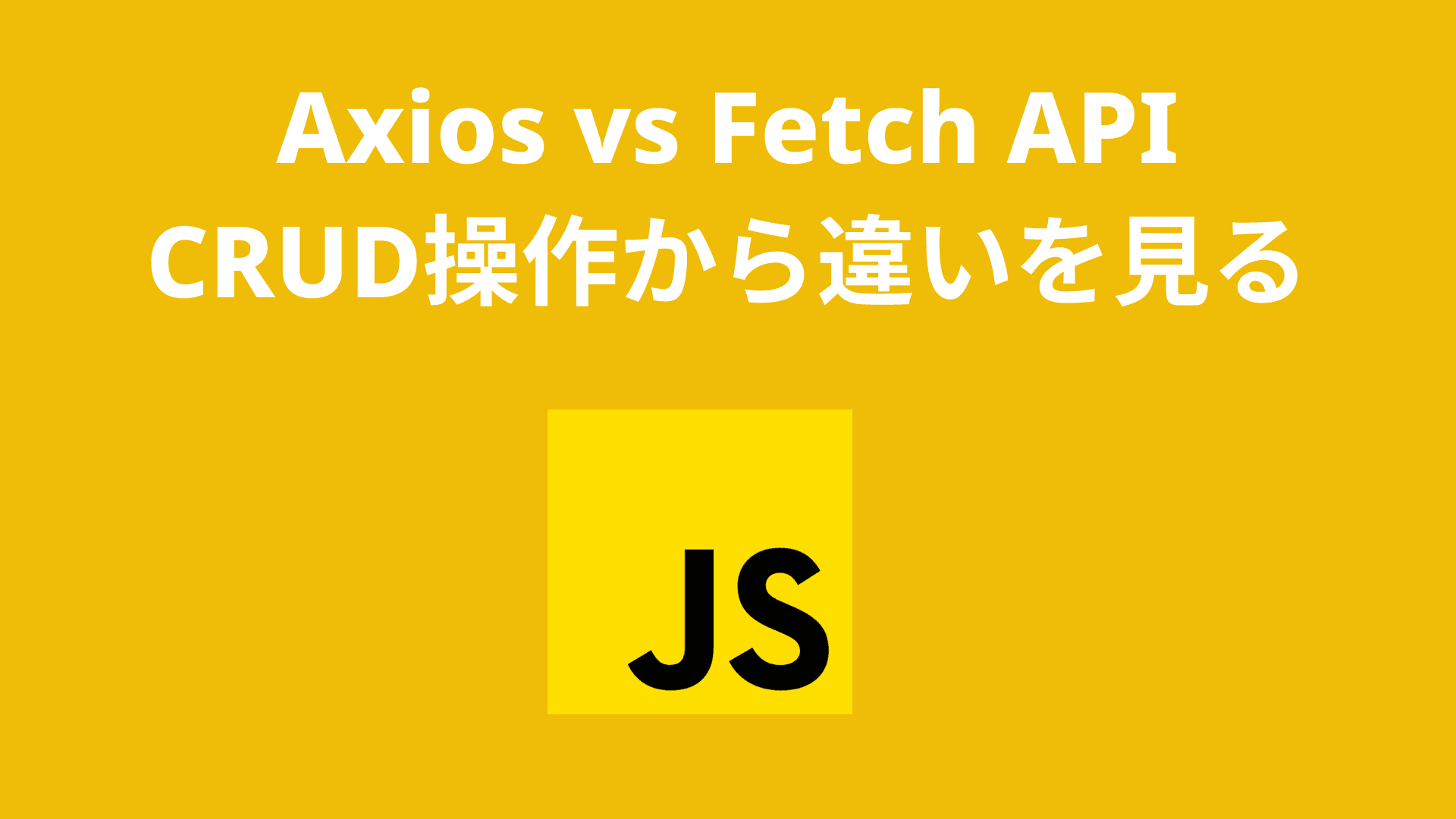
シェアしてね〜🤞
AxiosとFetch APIはhttp requestを行う技術
今回のテーマであるAxiosとFetch APIはどちらもhttp requestを行う技術ですが、
Axiosはjsライブラリで、Fetch APIはネイティブで実装されているという違いがあります。
この記事では、CRUD操作において、2つの違いを解説していきます
Axiosの使い方
まずは、Axiosの使い方についてCRUD操作を用いて解説していきます。
Axiosは、既存のHTTPクライアントの機能を簡単に利用できるようにし、さらに便利な機能や拡張性を提供することを目的として作られました。これは、従来のXMLHttpRequest(XHR)よりも使いやすい方法を提供するために作られました。
AxiosでのCreate操作
const data = { firstName : "Taro", lastName : "Yamada" }
const url = axios.post("http://localhost:3000/user/123", data)
//then=成功した場合の処理
.then(() => {
console.log(url)
})
//catch=エラー時の処理
.catch(err => {
console.log("err:", err);
});
AxiosでのRead操作
const url = axios.get("[http://localhost:3000/](http://localhost:3000/)")
.then(() => {
console.log("ステータスコード:", status);
})
.catch(err => {
console.log("err:", err);
});
AxiosでのUpdate操作
const data = { firstName : "Taro", lastName : "Qiita" }
const url = axios.put("http://localhost:3000/user/123",data)
.then(() => {
console.log('データ更新:',url)
})
.catch(err => {
console.log("err:", err);
});
AxiosでのDelete操作
const url = axios.delete("http://localhost:3000/user/123")
.then(() => {
console.log('削除ID:',url)
})
.catch(err => {
console.log("err:", err);
});
Fetch APIの使い方
FetchAPIはPromiseベースで、直感的でシンプルな構文を備えており、非同期操作ができます。また、リクエストやレスポンスをより細かく制御でき、新しい機能や拡張性も提供しています。
Fetch APIでのCreate操作
fetch("https://jsonplaceholder.typicode.com/posts", {
//リクエスト形式を指定
method: "POST",
//サーバに送信するデータを指定
body: JSON.stringify({
title: "Hello World",
body: "My POST request",
userId: 900,
}),
//サーバーにJSON形式で送信することを指定
headers: {
"Content-type": "application/json; charset=UTF-8",
},
})
.then((response) => response.json())
.then((json) => console.log(json));
Fetch APIでのRead操作
fetch("https://jsonplaceholder.typicode.com/posts")
.then((response) => response.json())
.then((json) => console.log(json));
Fetch APIでのUpdate操作
fetch("https://jsonplaceholder.typicode.com/posts/1", {
method: "PUT",
body: JSON.stringify({
id: 1,
title: "My PUT request",
body: "Updating the entire object",
userId: 1,
}),
headers: {
"Content-type": "application/json; charset=UTF-8",
},
})
.then((response) => response.json())
.then((json) => console.log(json));
Fetch APIでのDelete操作
//IDが3であるオブジェクトを削除
fetch("https://jsonplaceholder.typicode.com/posts/3", {
method: "DELETE",
});
AxiosとFetch APIの違い
AxiosとFetch APIは、HTTPリクエストを処理するために使用される2つの異なるアプローチです。以下に、両者の違いに関する簡単な説明を示します。
Axios
- Axiosは、ブラウザとNode.jsの両方で動作するHTTPクライアントライブラリ
- PromiseベースのAPIを提供し、非同期処理が可能
- 高度な機能(自動的なJSON変換、エラーハンドリングなど)が組み込まれており、直感的な使用が可能
Fetch API
- Fetch APIは、ブラウザに組み込まれているネイティブのJavaScript APIで、ネットワークリクエストを扱う
- FetchはPromiseベースであり、比較的シンプルな構文
- 追加の機能や設定を必要とせず、純粋なJavaScriptの機能
上記のように、CRUD操作の例では、Axiosは自動的にJSONデータを処理し、エラーハンドリングを提供するなど、使いやすいAPIを提供しています。
一方でFetch APIは、ネイティブのJavaScript APIであるため、一部の機能や設定を手動で行う必要があります。どちらを選択するかは、プロジェクトの要件や開発者の好みによります。
この記事のまとめ
- Axiosは自動的にJSONデータを処理し、エラーハンドリングを提供するなど初めての人に使いやすい
- Fetch APIは一部の機能や設定を手動で設定できるため、自由度が高い
シェアしてね〜🤞